Checkout.js Documentation
Securely collect, verify, and debit customer bank accounts now or later — all through a simple Paynote-hosted or embedded checkout.
Overview
Paynote Checkout.js is a simple, flexible checkout flow that lets you securely collect a customer's bank account information using Plaid — either embedded on your site or through a secure Paynote-hosted page.
You can use Checkout.js to:
- Debit a customer's bank account immediately (one-time payment)
- Collect and authorize a customer's bank details now, and debit them later (authorization-only)
All bank account information is securely verified through Plaid and sent directly to Paynote for processing — keeping your servers out of PCI scope.
Checkout.js supports:
- Embedded checkout via iframe
- Redirect checkout via a hosted Paynote page
- One-time debits
- Future debits after authorization
All customer bank data is tokenized and transmitted securely to Paynote for processing, keeping your platform out of PCI scope.
Have Questions or need help deciding?
Reach out to [email protected]
Environment Mode
- For testing environments, use
sandbox: true
- For live production environments, use
sandbox: false
Always test your full payment flow before going live.
Quick Start
1. Add the SDK to your HTML
<script type="text/javascript" src="https://developers.seamlesschex.com/docs/checkoutjs/sdk-min.js"></script>
2. Insert integration script
<script>
window.onload = function() {
var paynote = new PAYNOTE({
publicKey: 'your-public-key',
sandbox: true,
displayMethod: 'iframe', // or 'redirect'
paymentToken: 'pay_tok_' + Math.random(),
widgetContainerSelector: 'wrapper-pay-buttons'
});
paynote.render();
};
</script>
3. Replace 'your-public-key'
with your Paynote API key.
'your-public-key'
with your Paynote API key.Installation (SDK Setup)
Include the SDK:
<script type="text/javascript" src="https://developers.seamlesschex.com/docs/checkoutjs/sdk-min.js"></script>
Integration Methods
iframe Integration
Use iframe
to embed Checkout directly into your page.
displayMethod: 'iframe'
- Modal window opens over your checkout page.
- Supports custom event handling.
redirect Integration
Use redirect
to send customers to a Paynote-hosted page.
displayMethod: 'redirect'
- Hosted securely by Paynote.
- Customers are redirected back to your site after payment.
Configuration Options
This is a complete list of Checkout.js configuration options.
Checkout Details
Define the transaction details inside the checkout
object.
Key | Description | Default |
---|---|---|
totalValue | Payment amount to charge. (Required) | 0 |
currency | Currency of the payment. (Required) | USD |
description | Description of the transaction. (Required) | n/a |
items | Array of items purchased. Example: [{ title: '45 Units', price: 100 }] (Required) | n/a |
recurring | Recurring payment options (see below). | { billing_cycle: null, recurring: false } |
customerEmail | Customer email address. (Required) | n/a |
customerFirstName | Customer's first name. (Required) | n/a |
customerLastName | Customer's last name. (Required) | n/a |
companyName | Company name. (Optional) | n/a |
phone_number | Phone number, digits only. (Optional) | n/a |
Recurring Options
Example:
recurring: {
billing_cycle: 'month',
recurring: true,
num_of_payments: 3,
start_cycle: '2025-06-01'
}
Available billing_cycle
values:
day
week
bi-weekly
month
Set num_of_payments: null
for unlimited recurring charges.
Style Customization
Control button design with the style
object.
Example:
style: {
buttonClass: 'btn green-btn btn-block no-overflow',
buttonColor: '#00b660',
buttonLabelColor: '#ffffff',
buttonLabel: 'Pay Now'
}
Key | Description | Default |
---|---|---|
buttonColor | Background color of the button. | #00b660 |
buttonLabelColor | Color of the button text. | #ffffff |
buttonLabel | Text displayed inside the button. | Pay Now |
buttonClass | CSS class applied to the button. | btn btn-block btn-primary |
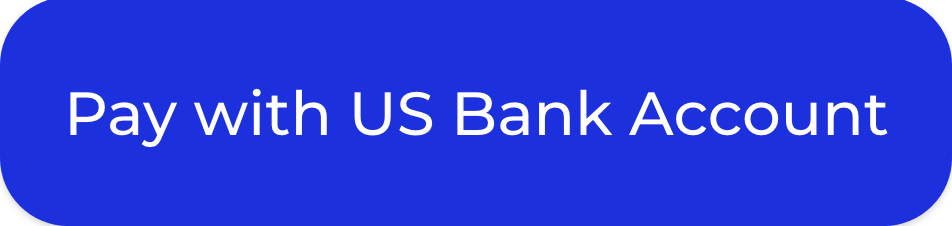
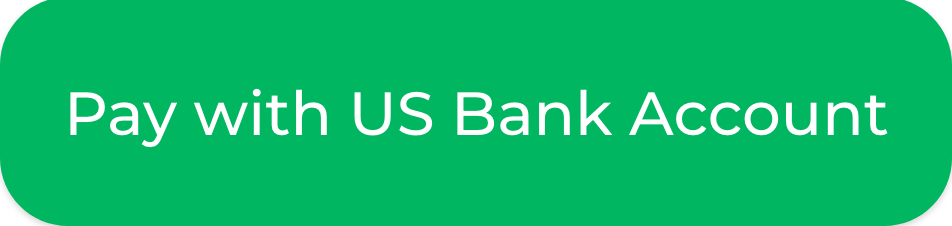
Lightbox Customization
Customize the modal window via the lightBox
object.
Example:
lightBox: {
title: 'Secure Payment',
subtitle: 'Complete Your Purchase',
logoUrl: 'https://yourwebsite.com/logo.png',
formButtonLabel: 'Pay Now',
show_cart_summary: false,
redirectUrl: 'https://yourwebsite.com/thank-you',
cancelUrl: 'https://yourwebsite.com/cancel'
}
Key | Description | Default |
---|---|---|
title | Title at the top of the form. | n/a |
subtitle | Subtitle under the title. | n/a |
logoUrl | Logo URL (must use HTTPS). | n/a |
formButtonLabel | Text inside the submit button. | PAY |
show_cart_summary | Whether to show order summary. | false |
redirectUrl | Redirect URL after success. | n/a |
cancelUrl | Redirect URL if canceled. | n/a |
Save Bank Account for Future Debits (Authorization-Only)
To securely store a customer's bank account details without processing an immediate payment, set the saveBankDetails
field to true
in your Checkout.js integration.
When saveBankDetails
is enabled:
- Paynote collects and securely stores the customer's bank information.
- No transaction is initiated during checkout by setting
totalvalue: 0
- You create and add a bank account to a customers profile
This setup is ideal for:
- Capturing Authorization from a customer
- Free Trial Models
- Delayed or future billing
✅ To skip immediate payment, you must also set authorizationOnly: true
alongside saveBankDetails: true
.
Example Usage
<script>
window.onload = function(e) {
var objRequestIframe = {
publicKey: 'pk_test_6ff46046-30af-41d9-bf58-11111111cd14',
sandbox: true,
saveBankDetails: true, // Securely store bank details
authorizationOnly: true, // Skip immediate payment
displayMethod: 'iframe',
paymentToken: 'pay_tok_SPECIMEN-' + Math.random(),
widgetContainerSelector: 'wrapper-pay-buttons',
style: {
buttonClass: 'btn green-btn btn-block no-overflow',
buttonColor: '#00b660',
buttonLabelColor: '#ffffff',
buttonLabel: 'Pay'
},
lightBox: {
title: 'Title Pay',
subtitle: 'Sub Title Pay',
logoUrl: "https://your.webSite.com/img/logo.png",
formButtonLabel: 'PAY',
show_cart_summary: false
},
checkout: {
totalValue: 50,
currency: 'USD',
description: 'Description',
items: [
{title: '45 Units', price: 50}
],
customerEmail: '[email protected]',
customerFirstName: 'Kelley',
customerLastName: 'Franecki'
},
onSuccess: function() {
yourCallBackFunction();
}
};
var paynoteIframe = new PAYNOTE(objRequestIframe);
paynoteIframe.render();
};
</script>
Important Notes
- No transaction is created at the time of collection.
- Stored bank accounts can be used for future payments through Paynote's APIs or invoicing tools.
- Ensure you have customer consent before initiating any future debits.
Event Handlers
Use event handlers with iframe
integrations only.
Example:
<script>
window.onload = function(e) {
var objRequestIframe = {
publicKey: 'your-public-key',
sandbox: true,
displayMethod: 'iframe',
paymentToken: 'pay_tok_' + Math.random(),
widgetContainerSelector: 'wrapper-pay-buttons',
onSuccess: function(data) {
console.log('Payment Success:', data);
},
onExit: function() {
console.log('User Exited Checkout');
},
onError: function(error) {
console.error('Checkout Error:', error);
},
onCancel: function() {
console.log('User Canceled Checkout');
}
};
var paynoteIframe = new PAYNOTE(objRequestIframe);
paynoteIframe.render();
};
</script>
Event | Description |
---|---|
onSuccess | Fired when the payment is completed successfully. |
onExit | Fired when the customer closes the checkout window. |
onError | Fired when an error occurs. |
onCancel | Fired when the customer cancels the payment. |
SDK
Our software development kit remove the need to handle sensitive data by enabling you to process payments and transactions in the same secure way as Frames and Paynote Checkout.js.
To set up basic integration include the following Paynote Checkout.js SDK in your HTML using a tag:
<script type="text/javascript" src="https://developers.seamlesschex.com/docs/checkoutjs/sdk-min.js"></script>
Updated 7 days ago